读书笔记《hands-on-full-stack-development-with-spring-boot-2-0-and-react》通过Reaction使用REST API
本章解释了使用 React 建立网络。我们将学习 Promise,它使异步代码更简洁、更易读。对于网络,我们将使用 fetch
库。作为示例,我们使用 GitHub REST API 来演示如何使用 React 使用 RESTful Web 服务。
在本章中,我们将看到以下内容:
- Using promises
- How to use Fetch
- How to make requests to the REST API
- How to handle responses from the REST API
- How to create a React app that consumes the REST API
在本书中,我们使用的是 Windows 操作系统,但所有工具都适用于 Linux 和 macOS,因为必须安装 Node.js 和 create-react-app
。 ;
处理异步操作的传统方法是使用回调函数来判断操作的成功或失败。调用回调函数之一,具体取决于调用的结果。下面的例子展示了使用回调函数的想法:
Promise 是一个表示异步操作结果的对象。使用 Promise 可以简化异步调用时的代码。 Promise 是非阻塞的。
- Pending: Initial state
- Fulfilled: Successful operation
- Rejected: Failed operation
使用 Promise,如果我们使用的 API 支持 Promise,我们就可以进行异步调用。在下一个示例中,异步调用完成,当返回响应时,执行 then
中的函数并将响应作为参数:
您可以将 thens
链接在一起,这意味着您可以一个接一个地运行多个异步操作:
您还可以使用 catch()
为 Promise 添加错误处理:
有一种更现代的方式来处理异步调用,使用 async
/await
,它是在 ECMAScript 2017 中引入的。不像 Promise 那样被浏览器广泛支持。 async
/await
实际上是基于 Promise 的。要使用 async
/await
,您必须定义一个 async
函数可以包含等待表达式。以下是使用 async
/await
的异步调用示例。如您所见,您可以使用与同步代码类似的方式编写代码:
对于错误处理,您可以使用 try...catch
和 async
/await
,如下例所示:
使用 Fetch API,您可以发出 web 请求。 Fetch API 的思想类似于传统的 XMLHttpRequest
,但 Fetch API 还支持 Promise,使其更易于使用。
Fetch API 提供了一个 fetch()
方法,该方法有一个强制参数,即您正在调用的资源的路径。在 Web 请求的情况下,它将是服务的 URL。对于返回 JSON 响应的简单 GET
方法调用,语法如下。 fetch()
方法返回一个包含响应的承诺。您可以使用 json()
方法从响应中解析 JSON 正文:
要使用其他 HTTP 方法,例如 POST
,您可以在 fetch
方法的第二个参数中定义它。第二个参数是您可以定义多个请求设置的对象。以下源代码使用 POST
方法发出请求:
您还可以在第二个参数中添加标题。以下 fetch
调用包含 'Content-Type' : 'application/json'
标头:
如果您必须在请求正文中发送 JSON 编码的数据,则语法如下:
您还可以使用其他库进行网络调用。一个非常流行的库是 axios
(https:// /github.com/axios/axios),您可以使用 npm 将其安装到您的 React 应用程序中。 axios 有一些好处,例如 JSON 数据的自动转换。以下代码显示了使用 axios
的示例调用:
axios
对不同的 HTTP 方法有自己的调用方法。例如,如果你想发出一个 DELETE
请求, axios
提供了 axios.delete
方法。
我们将通过两个示例使用 一些开放的 REST API。首先,我们将制作一个显示伦敦当前天气的 React 应用程序。 weather 是从 OpenWeatherMap ( https://openweathermap.org/)。您需要注册到 OpenWeatherMap 以获取 API 密钥。我们将使用免费帐户,因为这足以满足我们的需求。注册后,导航到您的帐户信息以找到 API 密钥选项卡。在那里,您将看到您的 React 天气应用程序所需的 API 密钥:
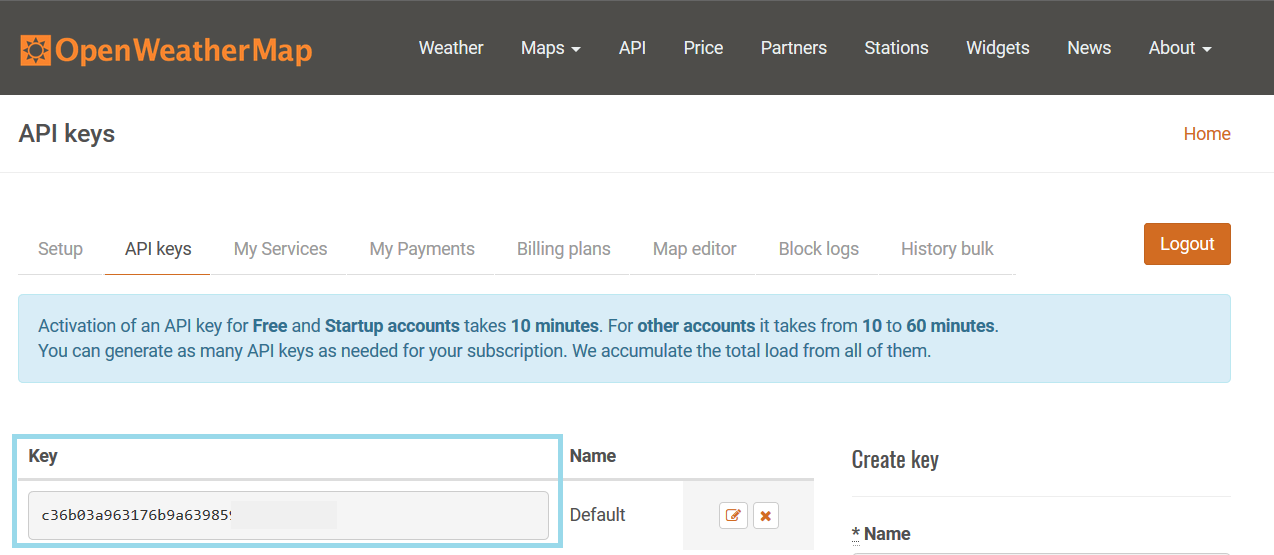
让我们用 create-react-app
创建一个新的 React 应用。打开您正在使用的 PowerShell 或其他终端,然后键入以下命令:
移动到 weatherApp
文件夹:
使用以下命令启动您的应用程序:
使用 VS Code 打开您的项目文件夹,然后在编辑器视图中打开 App.js
文件。删除 <div className="App"></div>
分隔符内的所有代码。现在您的源代码应如下所示:
Note
如果您已将 Reactjs 代码片段 安装到 VS Code,则可以通过键入 con
。典型的 React 方法有很多不同的快捷方式,例如
cdm
for componentDidMount()
。
首先,我们添加必要的构造函数和状态。我们将在我们的应用程序中显示温度、描述和天气图标,因此,我们定义了 三个 状态值。我们还将添加一个布尔状态来指示获取加载的状态。以下是构造函数的源代码:
当您使用 REST API 时,您应该首先检查响应以便能够从 JSON 数据中获取值。在以下示例中,您可以看到返回伦敦当前天气的地址。将地址复制到浏览器,可以看到 JSON 响应数据:
从响应中,您可以看到可以使用 main.temp
访问 temp
。 description
和 icon
在 weather
数组中,只有一个元素,我们可以使用 weather[0].description
和 weather[0].icon
来访问它:
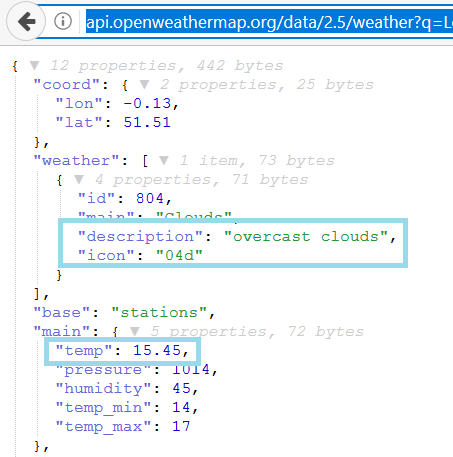
REST API 调用通过componentDidMount()
生命周期方法中的 fetch
完成。响应成功后,我们将天气数据保存到状态,并将 loading
状态改为false
。状态改变后,组件将被重新渲染。我们将在下一步中实现 render()
方法。以下是 componentDidMount()
方法的源码:
添加 componentDidMount()
方法后,请求在组件挂载时完成。我们可以使用 React Developer Tool 检查一切是否正确。在浏览器中打开您的应用并打开浏览器开发人员工具的 React
选项卡。现在您可以看到状态已使用响应中的值进行了更新。您还可以从 Network
选项卡检查请求状态是否为 200 OK:

最后,我们实现render()
方法来show 天气值.我们正在使用条件渲染,否则会出现错误,因为我们在第一次渲染调用中没有图像代码并且图像上传不会成功。要显示天气图标,我们必须在图标代码和 .png 之前添加
在图标代码之后。然后,我们可以将连接的图像 URL 设置为 http://openweathermap.org/img/w/
img
元素的 src
属性。温度和描述显示在段落元素中。 °C HTML 实体显示摄氏度符号:
现在你的应用程序应该准备好了。当您在浏览器中打开它时,它应该如下图所示:
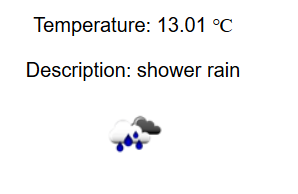
整个 App.js
文件的源代码如下:
在第二个示例中,我们将开始 使用 GitHub API 通过关键字获取存储库。使用与上一个示例相同的步骤,创建一个名为 restgithub
的新 React 应用程序。启动应用程序并使用 VS Code 打开项目文件夹。
从 App 中删除
文件,您的 <div className="App"></div>
divider 中的额外代码。 jsApp.js
代码应类似于以下示例代码:
GitHub REST API 的 URL 如下:
让我们通过在浏览器中输入 URL 并使用 react
关键字来检查 JSON 响应。从响应中,我们可以看到存储库作为名为 items
的 JSON 数组返回。从各个存储库中,我们将显示 full_name
和 html_url
值。我们将在表格中呈现数据,并使用 map
函数将值转换为表格行,如上一章所示:
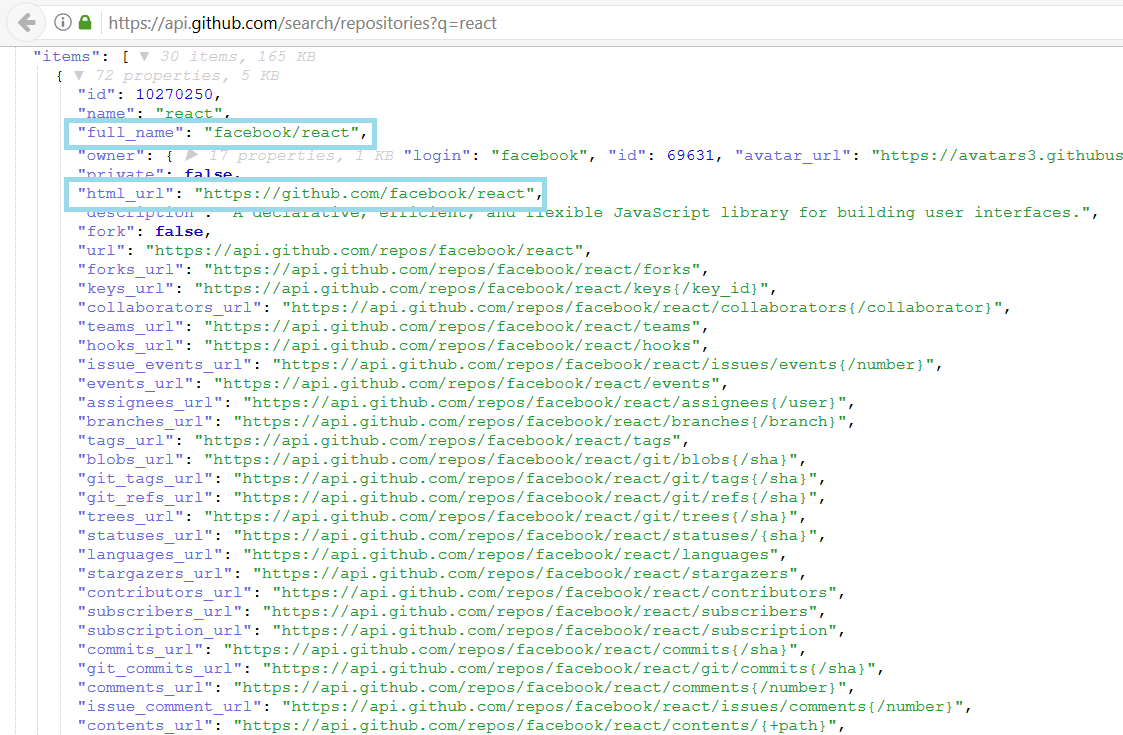
我们将使用来自用户输入的关键字进行 REST API 调用。因此,我们无法在 componentDidMount()
方法中调用 REST API,因为在那个阶段,我们没有可用的用户输入。实现这一点的一种方法是创建一个输入字段和按钮。用户在输入字段中键入关键字,按下按钮时完成 REST API 调用。我们需要两种状态,一种用于用户输入,另一种用于来自 JSON 响应的数据。以下是 构造函数
的源码。数据状态的类型是一个数组,因为存储库在响应中作为 JSON 数组返回:
接下来,我们将输入字段和按钮实现到 render()
方法中。我们还必须在输入字段中添加一个更改侦听器,以便能够将输入值保存到状态,称为 keyword
。该按钮有一个点击监听器,它调用函数 将使用给定的关键字进行 REST API 调用:
在 fetchData
函数中,我们连接了 url
和keyword
状态通过使用模板文字。然后我们将 items
数组从响应中保存到状态,称为data
。以下是fetchData
函数的源码:
在render
方法中,我们首先使用 map
函数对 数据< /code> 状态表行。
url
repository 将是链接元素的 href
:
以下屏幕截图显示了在 REST API 调用中使用 React 关键字时的最终应用程序:
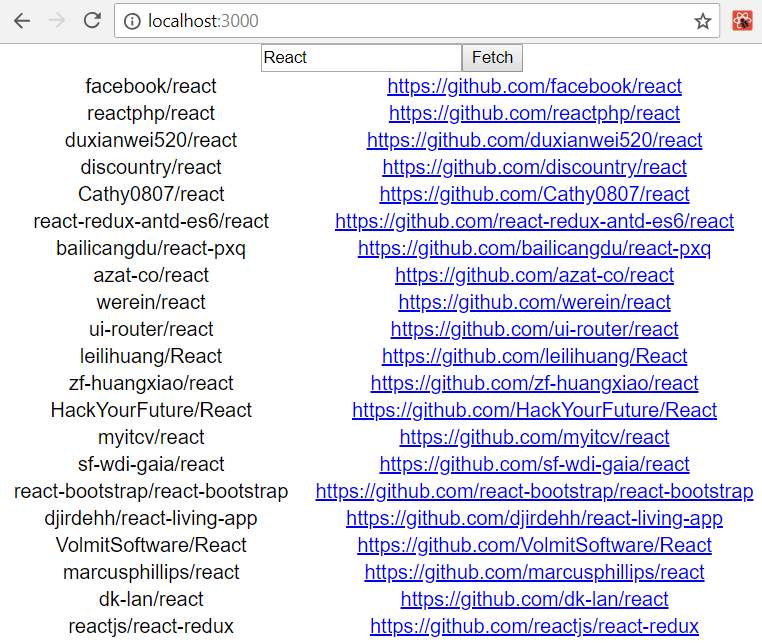
整个 App.js
文件的源代码如下所示:
Packt 还有其他学习 React 的好资源: