读书笔记《spring-security-3-x-cookbook》第 10 章使用 Spring Web 服务的 Spring Security
第 10 章使用 Spring Web 服务的 Spring Security
在本章中,我们将介绍:
在 RESTful Web 服务上应用 Spring Security
使用 cURL 工具的 Spring RESTful Web 服务的 Spring Security
将 Spring Security 与 Apache CXF RESTful 服务集成
将 Spring Security 与基于 Apache CXF SOAP 的 Web 服务集成
将 Spring Security 与 Apache Camel 集成
介绍
SOAP(简单对象访问协议)是基于 XML 的网络服务。它用于在 Web 服务之间传输请求和响应消息。
REST (Representational State Transfer) 是一种发送数据的方式通过 HTTP 协议作为 XML、文本或 JSON 文件。
在本节中,我们将 Spring Security 应用于 Web 服务。任何 Web 服务的正常流程是服务 WSDL 或 URL 将暴露给最终用户。在 Spring Security 的应用上,最终用户可以通过身份验证和授权使用服务。
在 RESTful Web 服务上应用 Spring Security
可以使用 XML、文本或 JSON 格式跨应用程序共享数据。 REST Web 服务被视为轻量级 Web 服务。
让我们应用 Spring Security 来访问 REST Web 服务,这样只有授权用户才能访问 RESTful Web 服务。由于使用 URL 访问 RESTful Web 服务并使用 HTTP 协议,我们可以轻松应用 URL 级别的安全性。此示例演示基于表单的身份验证。但用户也可以使用 BASIC 和 Digest Authentication。
下面是注释与Spring一起用于生成RESTful Web服务:
@PathVariable
@RequestMapping
@RequestMethod
准备好
使用 Spring Web 服务 API 创建 RESTful Web 服务
添加 Spring Security 依赖项
将 Spring 过滤器配置添加到
Web.xml
文件配置
application-security.xml
文件创建一个
AccessController
类来处理登录和注销操作在应用程序中配置 Spring Security 以对用户进行身份验证
怎么做...
以下是将 RESTful Web 服务与 Spring Security 集成的步骤:
让我们用
@PathVariable
创建一个BookController
类,如以下代码片段所示:创建
书籍
POJO带有@JsonAutoDetect
注解的类,如以下代码片段所示:创建 一个
AccessController
类来处理 登录和注销操作:配置
Application-security.xml
文件,如下代码片段所示:
这个怎么运作...
访问 URL:http://localhost:8080/booksservice/books/1
。这是基于 REST 的 URL, 使用 Spring Security 限制访问。当用户调用基于 REST 的 Web 服务 URL 时,Spring Security 会将用户重定向到登录页面。成功验证后,用户将被重定向到授权的基于 REST 的 Web 服务页面。
以下是使用 Spring Security 的基于 REST 的应用程序的工作流程。您将被重定向到登录页面,如以下屏幕截图所示:
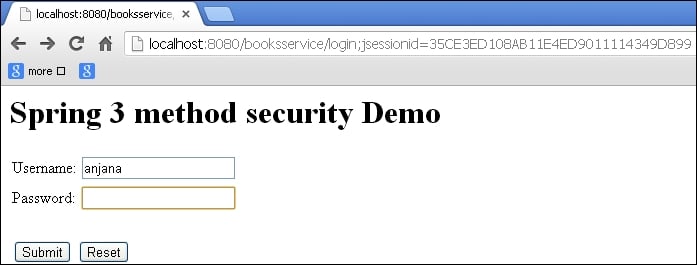
在身份验证和授权后,您将能够访问RESTful Web服务,如以下屏幕截图所示:

也可以看看
将 Spring Security 与 Apache CXF RESTful Web 服务集成 秘诀
将 Spring Security 与基于 Apache CXF SOAP 的 Web 服务集成 秘诀
将 Spring Security 与 Apache Camel 集成 秘诀
使用 cURL 工具的 Spring RESTful Web 服务的 Spring Security
在这个 示例中,我们明确使用了 Spring Security API 类和接口。我们将使用 curl
命令验证 RESTful Web 服务。使用 cURL 工具,您可以使用 URL 传输数据。它可用于测试身份验证。这是同一个图书服务示例,它具有一些明确的 Spring Security 相关 API 类,例如 AuthenticationEntryPoint
和 SimpleURLAuthenticationSuccessHandler
。在这里,目标是演示它们在 Spring Security 中的内部用法。
准备好
实现
AuthenticationEntryPoint
接口并在XML文件中配置扩展
SimpleUrlAuthenticationSuccessHandler
并在 XML 文件中配置配置
Application-security.xml
文件将安全相关过滤器添加到
Web.xml
文件下载适用于您的操作系统的 cURL 工具
怎么做...
以下是使用 AuthenticationEntryPoint
接口和 SimpleURLAuthenticationSuccessHandler
类应用 Spring Security 认证和授权机制的步骤:
这个怎么运作...
现在访问网址:http://localhost:8080/booksservice/books/1
让我们使用为我们提供 cookie 的 cURL 工具。 200 OK
消息暗示我们已通过身份验证。
cookie 存储在名为 mycookies.txt
的文件中。
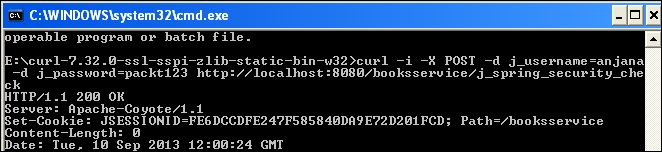
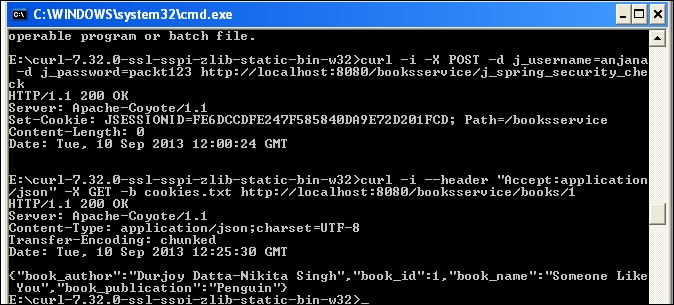
也可以看看
将 Spring Security 与 Apache CXF RESTful Web 服务集成 秘诀
将 Spring Security 与基于 Apache CXF SOAP 的 Web 服务集成 秘诀
将 Spring Security 与 Apache Camel 集成 秘诀
将 Spring Security 与 Apache CXF RESTful Web 服务集成
在 这部分让我们创建一个Apache CXF RESTful Web 服务。它是一个开源的 Web 服务框架。让我们在此演示中使用 BASIC 身份验证。
CXF 支持合约优先和合约最后的 Web 服务。它还支持 RESTful Web 服务。
让我们将 Spring Security 与 CXF 集成并授权 RESTful Web 服务。
准备好
将
cxf
依赖添加到pom
文件中使用 CXF 设置 RESTful Web 服务
配置
spring-security.xml
文件
怎么做...
以下是将 Spring Security 与 Apache CXF RESTful Web 服务集成的步骤:
这个怎么运作...
RESTful 服务 由 本示例中的CXF 框架提供。然后将应用程序与 Spring Security 集成,以便为 RESTful Web 服务提供安全的身份验证和授权模块。 Spring Security 过滤器链管理身份验证和授权过程。当您访问该服务时,系统会提示您登录,如以下屏幕截图所示。登录后可以查看 RESTful 数据。 Mozilla Firefox 浏览器将提示用户以文件格式下载数据。
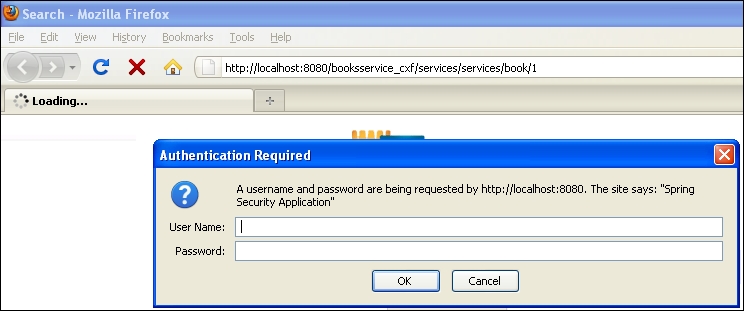
现在 访问 URL:http:// /localhost:8080/booksservice_cxf/services/services/book/1

也可以看看
将 Spring Security 与 Apache CXF RESTful Web 服务集成 秘诀
将 Spring Security 与 Apache Camel 集成 秘诀
将 Spring Security 与基于 Apache CXF SOAP 的 Web 服务集成
在这部分,让我们创建一个基于SOAP的web服务。我们将演示 Spring Security 与基于 Apache CXF SOAP 的 Web 服务的集成。
使用 Apache CXF 创建基于 SOAP 的 Web 服务已成为一个简单的过程。
准备好
怎么做...
以下是将基于 Apache CXF SOAP 的 Web 服务与 Spring Security 集成的步骤:
Book
POJO 有 getter 和 setter 方法。它还有一个参数化的构造函数。Book
POJO用于BookService
接口,提供Book 从客户端应用程序请求。
BookService
接口是 使用@WebService
创建的注释,在 中getBookDetails
是 WSDL 中的服务方法。BookServiceImpl
类是BookService
接口的实现类,使用@webservice
注释 包org.packt.cxf.service
。在
Cxf-servlet.xml< /code> 文件,我们注册Web服务接口和实现类。
在
Web.xml
文件中,我们参考cxf-servlet.xml
的位置并配置CXFSservlet
。
这个怎么运作...
在这部分我们演示了在网络服务上的基本身份验证。访问网址:http://localhost:8080/bookservice/
我们使用 CXF 框架创建了一个基于 SOAP 的 Web 服务。当用户访问 URL 时,预期的行为是授予对 WSDL 及其服务的访问权限。但是 Spring Security 会中断请求并为用户弹出一个登录对话框。认证成功后,用户可以访问 WSDL。
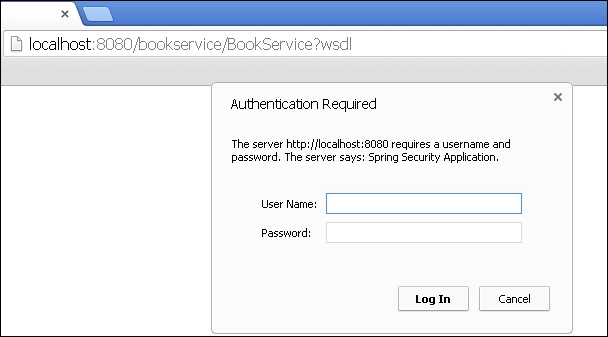
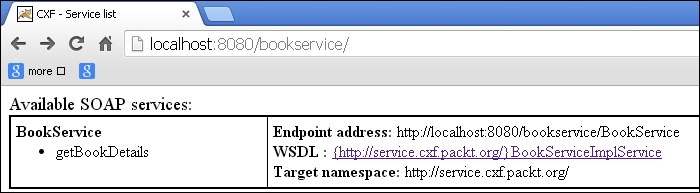
生成的 WSDL 可在以下 URL 获得:http://localhost:8080/bookservice/BookService?wsdl
也可以看看
将 Spring Security 与 Apache CXF RESTful Web 服务集成 秘诀
将 Spring Security 与 Apache Camel 集成 秘诀
将 Spring Security 与 Apache Camel 集成
Apache Camel 可用于定义路由和中介应用程序的 规则。 Spring Security 可以与 Apache Camel 一起使用来对路由器进行身份验证。 Spring Security 身份验证策略对象控制对路由器的访问。 Spring Security 身份验证策略对象包含角色信息并引用 Spring 身份验证管理器。您可以从网站下载源代码。
准备好
创建骆驼上下文
使用 XML 配置添加路由规则
在 Spring XML 文件中配置以下内容:
访问决策管理器
角色选民
身份验证管理器
用户详情服务
配置具有权限的身份验证策略对象
添加
camel-spring-security
依赖
怎么做...
这个怎么运作...
现在访问 URL:http://localhost:8080/apachecamel/editor
camel-context.xml
文件有路由规则; camel-context.xml
文件的位置与 一起在
来处理路由机制。 Web.xml
中配置CamelServlet<authorizationpolicy>
标签处理spring-security.xml
文件中配置的资源的认证和授权。 <spring-security:user-service>
标签包含在路由请求之前可以授予访问权限的用户和角色的详细信息。以下是 Apache Camel 使用 Spring Security 中断路由过程 的工作流程。用户具有以下两个角色之一的授权:EDITOR
或 AUTHOR
。
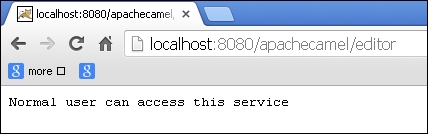
也可以看看
将 Spring Security 与 Apache CXF RESTful Web 服务集成 秘诀
将 Spring Security 与 Apache Camel 集成 秘诀